mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-07-14 17:59:38 -06:00
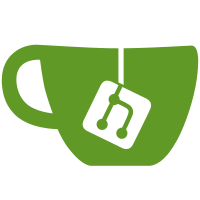
Keeps associated data together. It also eliminates the possibility of out parameters not being initialized properly. For example, consider the following example: -- some FramebufferManager implementation -- void FBMgrImpl::GetTargetSize(u32* width, u32* height) override { // Do nothing } -- somewhere else where the function is used -- u32 width, height; framebuffer_manager_instance->GetTargetSize(&width, &height); if (texture_width != width) <-- Uninitialized variable usage { ... } It makes it much more obvious to spot any initialization issues, because it requires something to be returned, as opposed to allowing an implementation to just not do anything.
35 lines
991 B
C++
35 lines
991 B
C++
// Copyright 2015 Dolphin Emulator Project
|
|
// Licensed under GPLv2+
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <utility>
|
|
|
|
#include "Common/CommonTypes.h"
|
|
#include "VideoCommon/FramebufferManagerBase.h"
|
|
|
|
class XFBSource : public XFBSourceBase
|
|
{
|
|
public:
|
|
void DecodeToTexture(u32 xfb_addr, u32 fb_width, u32 fb_height) override {}
|
|
void CopyEFB(float gamma) override {}
|
|
};
|
|
|
|
class FramebufferManager : public FramebufferManagerBase
|
|
{
|
|
public:
|
|
std::unique_ptr<XFBSourceBase> CreateXFBSource(unsigned int target_width,
|
|
unsigned int target_height,
|
|
unsigned int layers) override
|
|
{
|
|
return std::make_unique<XFBSource>();
|
|
}
|
|
|
|
std::pair<u32, u32> GetTargetSize() const override { return std::make_pair(0, 0); }
|
|
void CopyToRealXFB(u32 xfb_addr, u32 fb_stride, u32 fb_height, const EFBRectangle& source_rc,
|
|
float gamma = 1.0f) override
|
|
{
|
|
}
|
|
};
|