mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2024-11-15 05:47:56 -07:00
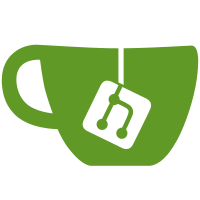
1. Avoided LoadLibrary() and FreeLibrary() in favor of using an existing LoadLibrary() because of an unresolved crash related to LoadLibrary() and nJoy. After several times of LoadLibrary() and FreeLibrary() of nJoy these seems to be some kind of memory corruption that brings up several error boxes from different places (not just nJoy) and then cause a crash. 2. Slowed down the moving of the pad initialization to InputCommon. I'm not against InputCommon at all, but I have to spend some more time with it before it works on Windows 3. Partly fixed a somewhat frequent failure of nJoy with SDL 1.3 that would give a strange DirectInput error message and cause the pads to not function. It's still present before the nJoy config windows is opened. It's only fixed on booting a game. git-svn-id: https://dolphin-emu.googlecode.com/svn/trunk@1991 8ced0084-cf51-0410-be5f-012b33b47a6e
93 lines
3.1 KiB
C
93 lines
3.1 KiB
C
//__________________________________________________________________________________________________
|
|
// Common pad plugin spec, version #1.0 maintained by F|RES
|
|
//
|
|
|
|
#ifndef _PAD_H_INCLUDED__
|
|
#define _PAD_H_INCLUDED__
|
|
|
|
#include "PluginSpecs.h"
|
|
|
|
#include "ExportProlog.h"
|
|
|
|
#define PAD_ERR_NONE 0
|
|
#define PAD_ERR_NO_CONTROLLER -1
|
|
#define PAD_ERR_NOT_READY -2
|
|
#define PAD_ERR_TRANSFER -3
|
|
|
|
#define PAD_USE_ORIGIN 0x0080
|
|
|
|
#define PAD_BUTTON_LEFT 0x0001
|
|
#define PAD_BUTTON_RIGHT 0x0002
|
|
#define PAD_BUTTON_DOWN 0x0004
|
|
#define PAD_BUTTON_UP 0x0008
|
|
#define PAD_TRIGGER_Z 0x0010
|
|
#define PAD_TRIGGER_R 0x0020
|
|
#define PAD_TRIGGER_L 0x0040
|
|
#define PAD_BUTTON_A 0x0100
|
|
#define PAD_BUTTON_B 0x0200
|
|
#define PAD_BUTTON_X 0x0400
|
|
#define PAD_BUTTON_Y 0x0800
|
|
#define PAD_BUTTON_START 0x1000
|
|
|
|
typedef void (*TLog)(const char* _pMessage);
|
|
|
|
typedef struct
|
|
{
|
|
HWND hWnd;
|
|
TLog pLog;
|
|
int padNumber;
|
|
} SPADInitialize;
|
|
|
|
typedef struct
|
|
{
|
|
unsigned short button; // Or-ed PAD_BUTTON_* and PAD_TRIGGER_* bits
|
|
unsigned char stickX; // 0 <= stickX <= 255
|
|
unsigned char stickY; // 0 <= stickY <= 255
|
|
unsigned char substickX; // 0 <= substickX <= 255
|
|
unsigned char substickY; // 0 <= substickY <= 255
|
|
unsigned char triggerLeft; // 0 <= triggerLeft <= 255
|
|
unsigned char triggerRight; // 0 <= triggerRight <= 255
|
|
unsigned char analogA; // 0 <= analogA <= 255
|
|
unsigned char analogB; // 0 <= analogB <= 255
|
|
bool MicButton; // HAX
|
|
signed char err; // one of PAD_ERR_* number
|
|
} SPADStatus;
|
|
|
|
/////////////////////////////////////////////////////////////////////////////////////////////////////
|
|
// I N T E R F A C E ///////////////////////////////////////////////////////////
|
|
|
|
// __________________________________________________________________________________________________
|
|
// Function:
|
|
// Purpose:
|
|
// input:
|
|
// output:
|
|
//
|
|
EXPORT void CALL PAD_GetStatus(u8 _numPAD, SPADStatus* _pPADStatus);
|
|
|
|
// __________________________________________________________________________________________________
|
|
// Function: Send keyboard input to the plugin
|
|
// Purpose:
|
|
// input: The key and if it's pressed or released
|
|
// output: None
|
|
//
|
|
EXPORT void CALL PAD_Input(u16 _Key, u8 _UpDown);
|
|
|
|
// __________________________________________________________________________________________________
|
|
// Function: PAD_Rumble
|
|
// Purpose: Pad rumble!
|
|
// input: PAD number, Command type (Stop=0, Rumble=1, Stop Hard=2) and strength of Rumble
|
|
// output: none
|
|
//
|
|
EXPORT void CALL PAD_Rumble(u8 _numPAD, unsigned int _uType, unsigned int _uStrength);
|
|
|
|
// __________________________________________________________________________________________________
|
|
// Function: PAD_GetAttachedPads
|
|
// Purpose: Get mask of attached pads (eg: controller 1 & 4 -> 0x9)
|
|
// input: none
|
|
// output: number of pads
|
|
//
|
|
EXPORT unsigned int CALL PAD_GetAttachedPads();
|
|
|
|
#include "ExportEpilog.h"
|
|
#endif
|