mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-07-13 09:19:32 -06:00
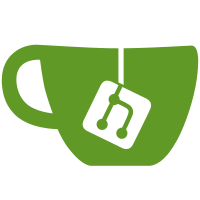
There were three distinct mechanisms for signaling breakpoint changes in DolphinQt, and the wiring had room for improvement. The behavior of these signals has been consolidated into the new `Host::PPCBreakpointsChanged` signal, which can be emitted from anywhere in DolphinQt to properly update breakpoints everywhere in DolphinQt. This improves a few things: - For the `CodeViewWidget` and `MemoryViewWidget`, signals no longer need to propagate through the `CodeWidget` and `MemoryWidget` (respectively) to reach their destination (incoming or outgoing). - For the `BreakpointWidget`, by self-triggering from its own signal, it no longer must manually call `Update()` after all of the emission sites. - For the `BranchWatchDialog`, it now has one less thing it must go through the `CodeWidget` for, which is a plus.
57 lines
1.5 KiB
C++
57 lines
1.5 KiB
C++
// Copyright 2015 Dolphin Emulator Project
|
|
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
|
|
#pragma once
|
|
|
|
#include <atomic>
|
|
|
|
#include <QObject>
|
|
|
|
// Singleton that talks to the Core via the interface defined in Core/Host.h.
|
|
// Because Host_* calls might come from different threads than the MainWindow,
|
|
// the Host class communicates with it via signals/slots only.
|
|
|
|
// Many of the Host_* functions are ignored, and some shouldn't exist.
|
|
class Host final : public QObject
|
|
{
|
|
Q_OBJECT
|
|
|
|
public:
|
|
~Host();
|
|
|
|
static Host* GetInstance();
|
|
|
|
bool GetRenderFocus();
|
|
bool GetRenderFullFocus();
|
|
bool GetRenderFullscreen();
|
|
bool GetGBAFocus();
|
|
bool GetTASInputFocus() const;
|
|
|
|
void SetMainWindowHandle(void* handle);
|
|
void SetRenderHandle(void* handle);
|
|
void SetRenderFocus(bool focus);
|
|
void SetRenderFullFocus(bool focus);
|
|
void SetRenderFullscreen(bool fullscreen);
|
|
void SetTASInputFocus(bool focus);
|
|
void ResizeSurface(int new_width, int new_height);
|
|
|
|
signals:
|
|
void RequestTitle(const QString& title);
|
|
void RequestStop();
|
|
void RequestRenderSize(int w, int h);
|
|
void UpdateDisasmDialog();
|
|
void PPCSymbolsChanged();
|
|
void PPCBreakpointsChanged();
|
|
|
|
private:
|
|
Host();
|
|
|
|
std::atomic<void*> m_render_handle{nullptr};
|
|
std::atomic<void*> m_main_window_handle{nullptr};
|
|
std::atomic<bool> m_render_to_main{false};
|
|
std::atomic<bool> m_render_focus{false};
|
|
std::atomic<bool> m_render_full_focus{false};
|
|
std::atomic<bool> m_render_fullscreen{false};
|
|
std::atomic<bool> m_tas_input_focus{false};
|
|
};
|