mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2024-11-15 13:57:57 -07:00
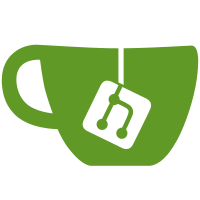
We can do this now that the x86-64 JIT supports PIE. JITIL is deliberately excluded from the GUI because it doesn't support PIE yet. (JITIL will be used if it's set in the INI, though.)
48 lines
1.1 KiB
C++
48 lines
1.1 KiB
C++
// Copyright 2015 Dolphin Emulator Project
|
|
// Licensed under GPLv2+
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <vector>
|
|
#include <wx/arrstr.h>
|
|
#include <wx/panel.h>
|
|
|
|
class wxButton;
|
|
class wxCheckBox;
|
|
class wxChoice;
|
|
class wxRadioBox;
|
|
|
|
class GeneralConfigPane final : public wxPanel
|
|
{
|
|
public:
|
|
GeneralConfigPane(wxWindow* parent, wxWindowID id);
|
|
|
|
private:
|
|
void InitializeGUI();
|
|
void LoadGUIValues();
|
|
void BindEvents();
|
|
|
|
void OnDualCoreCheckBoxChanged(wxCommandEvent&);
|
|
void OnCheatCheckBoxChanged(wxCommandEvent&);
|
|
void OnForceNTSCJCheckBoxChanged(wxCommandEvent&);
|
|
void OnThrottlerChoiceChanged(wxCommandEvent&);
|
|
void OnCPUEngineRadioBoxChanged(wxCommandEvent&);
|
|
void OnAnalyticsCheckBoxChanged(wxCommandEvent&);
|
|
void OnAnalyticsNewIdButtonClick(wxCommandEvent&);
|
|
|
|
wxArrayString m_throttler_array_string;
|
|
wxArrayString m_cpu_engine_array_string;
|
|
|
|
wxCheckBox* m_dual_core_checkbox;
|
|
wxCheckBox* m_cheats_checkbox;
|
|
wxCheckBox* m_force_ntscj_checkbox;
|
|
|
|
wxCheckBox* m_analytics_checkbox;
|
|
wxButton* m_analytics_new_id;
|
|
|
|
wxChoice* m_throttler_choice;
|
|
|
|
wxRadioBox* m_cpu_engine_radiobox;
|
|
};
|