mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-07-05 13:29:33 -06:00
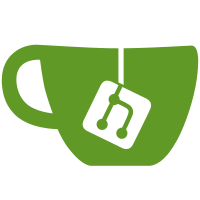
Analytics: - Incorporated fix to allow the full set of analytics that was recommended by spotlightishere BuildMacOSUniversalBinary: - The x86_64 slice for a universal binary is now built for 10.12 - The universal binary build script now can be configured though command line options instead of modifying the script itself. - os.system calls were replaced with equivalent subprocess calls - Formatting was reworked to be more PEP 8 compliant - The script was refactored to make it more modular - The com.apple.security.cs.disable-library-validation entitlement was removed Memory Management: - Changed the JITPageWrite*Execute*() functions to incorporate support for nesting Other: - Fixed several small lint errors - Fixed doc and formatting mistakes - Several small refactors to make things clearer
27 lines
896 B
C++
27 lines
896 B
C++
// Copyright 2008 Dolphin Emulator Project
|
|
// Licensed under GPLv2+
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <cstddef>
|
|
#include <string>
|
|
|
|
namespace Common
|
|
{
|
|
void* AllocateExecutableMemory(size_t size);
|
|
// Allows a thread to write to executable memory, but not execute the data.
|
|
void JITPageWriteEnableExecuteDisable();
|
|
// Allows a thread to execute memory allocated for execution, but not write to it.
|
|
void JITPageWriteDisableExecuteEnable();
|
|
void* AllocateMemoryPages(size_t size);
|
|
void FreeMemoryPages(void* ptr, size_t size);
|
|
void* AllocateAlignedMemory(size_t size, size_t alignment);
|
|
void FreeAlignedMemory(void* ptr);
|
|
void ReadProtectMemory(void* ptr, size_t size);
|
|
void WriteProtectMemory(void* ptr, size_t size, bool executable = false);
|
|
void UnWriteProtectMemory(void* ptr, size_t size, bool allowExecute = false);
|
|
size_t MemPhysical();
|
|
|
|
} // namespace Common
|