mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-06-30 10:59:36 -06:00
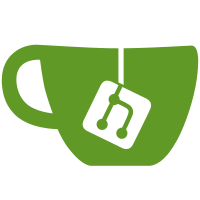
This works around Linux drivers for DS4 (Playstation 4) controllers splitting the device into three separate event nodes which makes configuration difficult. To prevent collisions of input names in combined devices more descriptive names are now used when possible.
102 lines
1.9 KiB
C++
102 lines
1.9 KiB
C++
// Copyright 2015 Dolphin Emulator Project
|
|
// Licensed under GPLv2+
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <libevdev/libevdev.h>
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
#include "InputCommon/ControllerInterface/ControllerInterface.h"
|
|
|
|
namespace ciface::evdev
|
|
{
|
|
void Init();
|
|
void PopulateDevices();
|
|
void Shutdown();
|
|
|
|
class evdevDevice : public Core::Device
|
|
{
|
|
private:
|
|
class Effect : public Core::Device::Output
|
|
{
|
|
public:
|
|
Effect(int fd);
|
|
~Effect();
|
|
void SetState(ControlState state) override;
|
|
|
|
protected:
|
|
virtual bool UpdateParameters(ControlState state) = 0;
|
|
|
|
ff_effect m_effect = {};
|
|
|
|
static constexpr int DISABLED_EFFECT_TYPE = 0;
|
|
|
|
private:
|
|
void UpdateEffect();
|
|
|
|
int const m_fd;
|
|
};
|
|
|
|
class ConstantEffect : public Effect
|
|
{
|
|
public:
|
|
ConstantEffect(int fd);
|
|
bool UpdateParameters(ControlState state) override;
|
|
std::string GetName() const override;
|
|
};
|
|
|
|
class PeriodicEffect : public Effect
|
|
{
|
|
public:
|
|
PeriodicEffect(int fd, u16 waveform);
|
|
bool UpdateParameters(ControlState state) override;
|
|
std::string GetName() const override;
|
|
};
|
|
|
|
class RumbleEffect : public Effect
|
|
{
|
|
public:
|
|
enum class Motor : u8
|
|
{
|
|
Weak,
|
|
Strong,
|
|
};
|
|
|
|
RumbleEffect(int fd, Motor motor);
|
|
bool UpdateParameters(ControlState state) override;
|
|
std::string GetName() const override;
|
|
|
|
private:
|
|
const Motor m_motor;
|
|
};
|
|
|
|
public:
|
|
void UpdateInput() override;
|
|
bool IsValid() const override;
|
|
|
|
~evdevDevice();
|
|
|
|
// Return true if node was "interesting".
|
|
bool AddNode(std::string devnode, int fd, libevdev* dev);
|
|
|
|
const char* GetUniqueID() const;
|
|
|
|
std::string GetName() const override { return m_name; }
|
|
std::string GetSource() const override { return "evdev"; }
|
|
|
|
private:
|
|
std::string m_name;
|
|
|
|
struct Node
|
|
{
|
|
std::string devnode;
|
|
int fd;
|
|
libevdev* device;
|
|
};
|
|
|
|
std::vector<Node> m_nodes;
|
|
};
|
|
} // namespace ciface::evdev
|