mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2024-11-15 05:47:56 -07:00
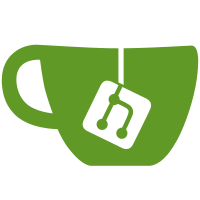
Achievement badges/icons are refactored into the type CustomTextureData::ArraySlice::Level as that is the data type images loaded from the filesystem will be. This includes everything that uses the badges in the Qt UI and OnScreenDisplay, and similarly removes the OSD::Icon type because Level already contains that information.
38 lines
994 B
C++
38 lines
994 B
C++
// Copyright 2023 Dolphin Emulator Project
|
|
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
|
|
#pragma once
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
#include "Common/CommonTypes.h"
|
|
#include "VideoCommon/TextureConfig.h"
|
|
|
|
namespace VideoCommon
|
|
{
|
|
class CustomTextureData
|
|
{
|
|
public:
|
|
struct ArraySlice
|
|
{
|
|
struct Level
|
|
{
|
|
std::vector<u8> data;
|
|
AbstractTextureFormat format = AbstractTextureFormat::RGBA8;
|
|
u32 width = 0;
|
|
u32 height = 0;
|
|
u32 row_length = 0;
|
|
};
|
|
std::vector<Level> m_levels;
|
|
};
|
|
std::vector<ArraySlice> m_slices;
|
|
};
|
|
|
|
bool LoadDDSTexture(CustomTextureData* texture, const std::string& filename);
|
|
bool LoadDDSTexture(CustomTextureData::ArraySlice::Level* level, const std::string& filename,
|
|
u32 mip_level);
|
|
bool LoadPNGTexture(CustomTextureData::ArraySlice::Level* level, const std::string& filename);
|
|
bool LoadPNGTexture(CustomTextureData::ArraySlice::Level* level, const std::vector<u8>& buffer);
|
|
} // namespace VideoCommon
|