mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2024-11-15 13:57:57 -07:00
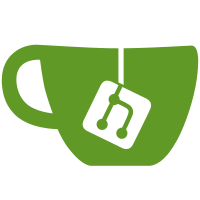
long become wxWidgets 2.9.2, which in turn is expected to be the last 2.9 release before the 3.0 stable release. Since the full wxWidgets distribution is rather large, I have imported only the parts that we use, on a subdirectory basis: art include/wx/*.* include/wx/aui include/wx/cocoa include/wx/generic include/wx/gtk include/wx/meta include/wx/msw include/wx/osx include/wx/persist include/wx/private include/wx/protocol include/wx/unix src/aui src/common src/generic src/gtk src/msw src/osx src/unix git-svn-id: https://dolphin-emu.googlecode.com/svn/trunk@7380 8ced0084-cf51-0410-be5f-012b33b47a6e
118 lines
3.2 KiB
C++
118 lines
3.2 KiB
C++
///////////////////////////////////////////////////////////////////////////////
|
|
// Name: wx/osx/evtloop.h
|
|
// Purpose: simply forwards to wx/osx/carbon/evtloop.h or
|
|
// wx/osx/cocoa/evtloop.h for consistency with the other Mac
|
|
// headers
|
|
// Author: Vadim Zeitlin
|
|
// Modified by:
|
|
// Created: 2006-01-12
|
|
// RCS-ID: $Id: evtloop.h 65680 2010-09-30 11:44:45Z VZ $
|
|
// Copyright: (c) 2006 Vadim Zeitlin <vadim@wxwindows.org>
|
|
// Licence: wxWindows licence
|
|
///////////////////////////////////////////////////////////////////////////////
|
|
|
|
#ifndef _WX_OSX_EVTLOOP_H_
|
|
#define _WX_OSX_EVTLOOP_H_
|
|
|
|
DECLARE_WXOSX_OPAQUE_CFREF( CFRunLoop );
|
|
DECLARE_WXOSX_OPAQUE_CFREF( CFRunLoopObserver );
|
|
|
|
class WXDLLIMPEXP_BASE wxCFEventLoop : public wxEventLoopBase
|
|
{
|
|
public:
|
|
wxCFEventLoop();
|
|
virtual ~wxCFEventLoop();
|
|
|
|
// enters a loop calling OnNextIteration(), Pending() and Dispatch() and
|
|
// terminating when Exit() is called
|
|
virtual int Run();
|
|
|
|
// sets the "should exit" flag and wakes up the loop so that it terminates
|
|
// soon
|
|
virtual void Exit(int rc = 0);
|
|
|
|
// return true if any events are available
|
|
virtual bool Pending() const;
|
|
|
|
// dispatch a single event, return false if we should exit from the loop
|
|
virtual bool Dispatch();
|
|
|
|
// same as Dispatch() but doesn't wait for longer than the specified (in
|
|
// ms) timeout, return true if an event was processed, false if we should
|
|
// exit the loop or -1 if timeout expired
|
|
virtual int DispatchTimeout(unsigned long timeout);
|
|
|
|
// implement this to wake up the loop: usually done by posting a dummy event
|
|
// to it (can be called from non main thread)
|
|
virtual void WakeUp();
|
|
|
|
virtual bool YieldFor(long eventsToProcess);
|
|
|
|
#if wxUSE_EVENTLOOP_SOURCE
|
|
virtual wxEventLoopSource *
|
|
AddSourceForFD(int fd, wxEventLoopSourceHandler *handler, int flags);
|
|
#endif // wxUSE_EVENTLOOP_SOURCE
|
|
|
|
void ObserverCallBack(CFRunLoopObserverRef observer, int activity);
|
|
|
|
protected:
|
|
// get the currently executing CFRunLoop
|
|
virtual CFRunLoopRef CFGetCurrentRunLoop() const;
|
|
|
|
virtual int DoDispatchTimeout(unsigned long timeout);
|
|
|
|
virtual void DoRun();
|
|
|
|
virtual void DoStop();
|
|
|
|
// should we exit the loop?
|
|
bool m_shouldExit;
|
|
|
|
// the loop exit code
|
|
int m_exitcode;
|
|
|
|
// cfrunloop
|
|
CFRunLoopRef m_runLoop;
|
|
|
|
// runloop observer
|
|
CFRunLoopObserverRef m_runLoopObserver;
|
|
|
|
private:
|
|
// process all already pending events and dispatch a new one (blocking
|
|
// until it appears in the event queue if necessary)
|
|
//
|
|
// returns the return value of DoDispatchTimeout()
|
|
int DoProcessEvents();
|
|
};
|
|
|
|
#if wxUSE_GUI
|
|
|
|
#ifdef __WXOSX_COCOA__
|
|
#include "wx/osx/cocoa/evtloop.h"
|
|
#else
|
|
#include "wx/osx/carbon/evtloop.h"
|
|
#endif
|
|
|
|
class WXDLLIMPEXP_FWD_CORE wxWindow;
|
|
class WXDLLIMPEXP_FWD_CORE wxNonOwnedWindow;
|
|
|
|
class WXDLLIMPEXP_CORE wxModalEventLoop : public wxGUIEventLoop
|
|
{
|
|
public:
|
|
wxModalEventLoop(wxWindow *modalWindow);
|
|
wxModalEventLoop(WXWindow modalNativeWindow);
|
|
|
|
protected:
|
|
virtual void DoRun();
|
|
|
|
virtual void DoStop();
|
|
|
|
// (in case) the modal window for this event loop
|
|
wxNonOwnedWindow* m_modalWindow;
|
|
WXWindow m_modalNativeWindow;
|
|
};
|
|
|
|
#endif // wxUSE_GUI
|
|
|
|
#endif // _WX_OSX_EVTLOOP_H_
|