mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2025-07-15 10:19:35 -06:00
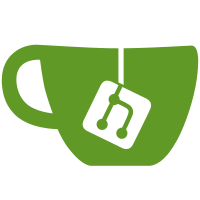
Previously, the Qt frontend would initialize the controller interface on starting, resulting in the cursor position being relative to the main window, instead of the render window.
65 lines
1.6 KiB
C++
65 lines
1.6 KiB
C++
// Copyright 2010 Dolphin Emulator Project
|
|
// Licensed under GPLv2+
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <atomic>
|
|
#include <functional>
|
|
#include <memory>
|
|
#include <mutex>
|
|
#include <vector>
|
|
|
|
#include "Common/WindowSystemInfo.h"
|
|
#include "InputCommon/ControllerInterface/Device.h"
|
|
|
|
// enable disable sources
|
|
#ifdef _WIN32
|
|
#define CIFACE_USE_XINPUT
|
|
#define CIFACE_USE_DINPUT
|
|
#endif
|
|
#if defined(HAVE_X11) && HAVE_X11
|
|
#define CIFACE_USE_XLIB
|
|
#endif
|
|
#if defined(__APPLE__)
|
|
#define CIFACE_USE_OSX
|
|
#endif
|
|
#if defined(HAVE_LIBEVDEV) && defined(HAVE_LIBUDEV)
|
|
#define CIFACE_USE_EVDEV
|
|
#endif
|
|
#if defined(USE_PIPES)
|
|
#define CIFACE_USE_PIPES
|
|
#endif
|
|
|
|
//
|
|
// ControllerInterface
|
|
//
|
|
// Some crazy shit I made to control different device inputs and outputs
|
|
// from lots of different sources, hopefully more easily.
|
|
//
|
|
class ControllerInterface : public ciface::Core::DeviceContainer
|
|
{
|
|
public:
|
|
ControllerInterface() : m_is_init(false) {}
|
|
void Initialize(const WindowSystemInfo& wsi);
|
|
void ChangeWindow(void* hwnd);
|
|
void RefreshDevices();
|
|
void Shutdown();
|
|
void AddDevice(std::shared_ptr<ciface::Core::Device> device);
|
|
void RemoveDevice(std::function<bool(const ciface::Core::Device*)> callback);
|
|
bool IsInit() const { return m_is_init; }
|
|
void UpdateInput();
|
|
|
|
void RegisterDevicesChangedCallback(std::function<void(void)> callback);
|
|
void InvokeDevicesChangedCallbacks() const;
|
|
|
|
private:
|
|
std::vector<std::function<void()>> m_devices_changed_callbacks;
|
|
mutable std::mutex m_callbacks_mutex;
|
|
bool m_is_init;
|
|
std::atomic<bool> m_is_populating_devices{false};
|
|
WindowSystemInfo m_wsi;
|
|
};
|
|
|
|
extern ControllerInterface g_controller_interface;
|