mirror of
https://github.com/dolphin-emu/dolphin.git
synced 2024-11-15 13:57:57 -07:00
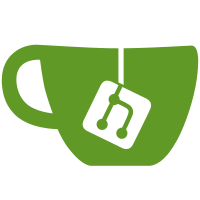
Now that MainNoGUI is properly architected and GLX doesn't need to sometimes craft its own windows sometimes which we have to thread back into MainNoGUI, we don't need to thread the window handle that GLX creates at all. This removes the reference to pass back here, and the g_pWindowHandle always be the same as the window returned by Host_GetRenderHandle(). A future cleanup could remove g_pWindowHandle entirely.
62 lines
1.6 KiB
C++
62 lines
1.6 KiB
C++
#pragma once
|
|
|
|
#include <string>
|
|
#include "VideoCommon/VideoBackendBase.h"
|
|
|
|
namespace MMIO { class Mapping; }
|
|
|
|
namespace SW
|
|
{
|
|
|
|
class VideoSoftware : public VideoBackend
|
|
{
|
|
bool Initialize(void *window_handle) override;
|
|
void Shutdown() override;
|
|
|
|
std::string GetName() const override;
|
|
std::string GetDisplayName() const override;
|
|
|
|
void EmuStateChange(EMUSTATE_CHANGE newState) override;
|
|
|
|
void RunLoop(bool enable) override;
|
|
|
|
void ShowConfig(void* parent) override;
|
|
|
|
void Video_Prepare() override;
|
|
void Video_Cleanup() override;
|
|
|
|
void Video_EnterLoop() override;
|
|
void Video_ExitLoop() override;
|
|
void Video_BeginField(u32, u32, u32) override;
|
|
void Video_EndField() override;
|
|
|
|
u32 Video_AccessEFB(EFBAccessType, u32, u32, u32) override;
|
|
u32 Video_GetQueryResult(PerfQueryType type) override;
|
|
|
|
void Video_AddMessage(const std::string& msg, unsigned int milliseconds) override;
|
|
void Video_ClearMessages() override;
|
|
bool Video_Screenshot(const std::string& filename) override;
|
|
|
|
int Video_LoadTexture(char *imagedata, u32 width, u32 height);
|
|
void Video_DeleteTexture(int texID);
|
|
void Video_DrawTexture(int texID, float *coords);
|
|
|
|
void Video_SetRendering(bool bEnabled) override;
|
|
|
|
void Video_GatherPipeBursted() override;
|
|
bool Video_IsPossibleWaitingSetDrawDone() override;
|
|
|
|
void RegisterCPMMIO(MMIO::Mapping* mmio, u32 base) override;
|
|
|
|
void UpdateFPSDisplay(const std::string&) override;
|
|
unsigned int PeekMessages() override;
|
|
|
|
void PauseAndLock(bool doLock, bool unpauseOnUnlock=true) override;
|
|
void DoState(PointerWrap &p) override;
|
|
|
|
public:
|
|
void CheckInvalidState() override;
|
|
};
|
|
|
|
}
|